Pyramid Pattern no. 8 using c code
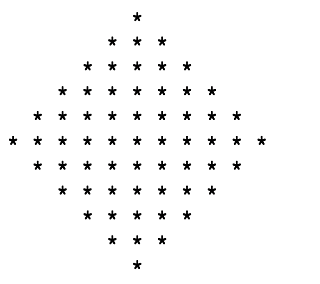
Pyramid Pattern no. 8 using c code /* To print Pyramid Pattern shown below */ #include<stdio.h> int main() { int i,j,n; printf("Enter no. of lines: "); scanf( "%d",&n); //for loop for upper pyramid //for loop for number of lines for(i=1;i<=n;i++) { //for loop for spaces for(j=1;j<=n-i;j++) { printf(" "); } //for loop for stars in first half for(j=1;j<=i;j++) { printf("* "); } //for loop for stars in second half for(j=1;j<i;j++) { printf("* "); } printf("\n"); } //for loop for lower pyramid //for loop for number of lines for(i=1;i<=n-1;i++) { //for loop for spaces for(j=1;j<=i;j++) { printf(" "); } //for loop for stars in first half for(j=1;j<=n-i;j++) { printf("* "); } //for loop for stars in second half for(j=1;j<n-i;j++) { printf("* "); } printf("\n"); } return 0; }